Explore

@Jenny
5
Children's Voice Over

@colinstark
5
Creative Director

Scarlett
Business Founder

@jordanruncie_
5
Production Assistant

Christina
Jewelry Shop Owner
Find the rightfreelanceservice, right away
Popular:
Trusted by:
Popular services
The best part? Everything.
Stick to your budget
Find the right service for every price point. No hourly rates, just project-based pricing.
Get quality work done quickly
Hand your project over to a talented freelancer in minutes, get long-lasting results.
Pay when you're happy
Upfront quotes mean no surprises. Payments only get released when you approve.
Count on 24/7 support
Our round-the-clock support team is available to help anytime, anywhere.
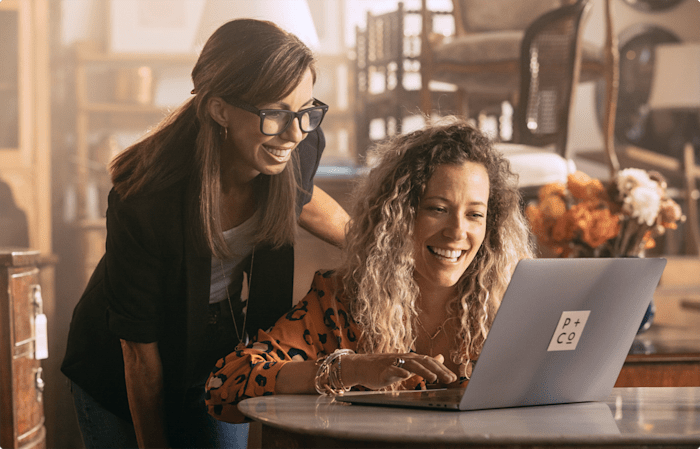